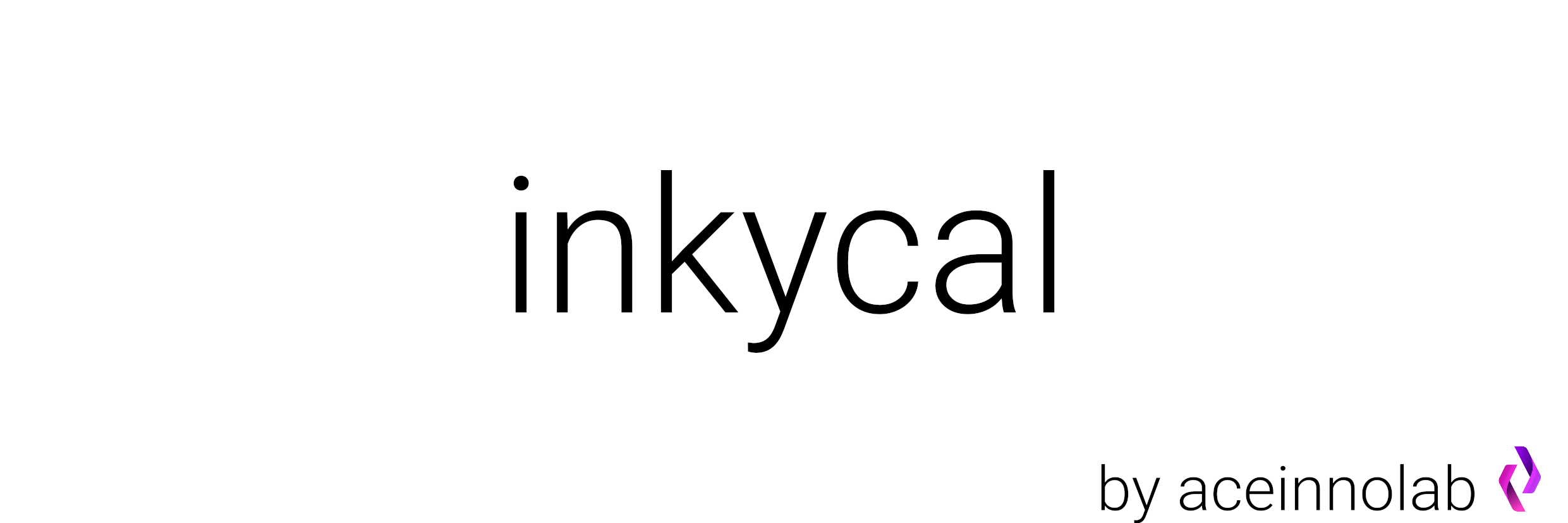
Inkycal
Main class for inkycal Project Copyright by aceinnolab
- class inkycal.main.Inkycal(settings_path: str = None, render: bool = True, use_pi_sugar: bool = False, shutdown_after_run: bool = False)
Inkycal main class
Main class of Inkycal, test and run the main Inkycal program.
- Args:
settings_path = str -> the full path to your settings.json file if no path is given, tries looking for settings file in /boot folder.
render = bool (True/False) -> show the image on the epaper display?
- Attributes:
optimize = True/False. Reduce number of colours on the generated image to improve rendering on E-Papers. Set this to False for 9.7” E-Paper.
- calibrate(cycles=3)
Calibrate the E-Paper display
Uses the Display class to calibrate the display with the default of 3 cycles. After a refresh cycle, a new image is generated and shown.
- countdown(interval_mins: int = None) int
Returns the remaining time in seconds until the next display update based on the interval.
- Args:
- interval_mins (int): The interval in minutes for the update. If none is given, the value
from the settings file is used.
- Returns:
int: The remaining time in seconds until the next update.
- dry_run()
Tests if Inkycal can run without issues.
Attempts to import module names from settings file. Loads the config for each module and initializes the module. Tries to run the module and checks if the images could be generated correctly.
Generated images can be found in the /images folder of Inkycal.
- process_module(number) bool
Process individual module to generate images and handle exceptions.
- async run(run_once=False)
Runs main program in nonstop mode or a single iteration based on the run_once flag.
- Args:
- run_once (bool): If True, runs the updating process once and stops. If False,
runs indefinitely.
Uses an infinity loop to run Inkycal nonstop or a single time based on run_once. Inkycal generates the image from all modules, assembles them in one image, refreshes the E-Paper and then sleeps until the next scheduled update or exits.
Display
Display class for inkycal
Creates an instance of the driver for the selected E-Paper model and allows rendering images and calibrating the E-Paper display
- Args:
epaper_model: The name of your E-Paper model.
Custom functions
Inkycal custom-functions for ease-of-use
Copyright by aceinnolab
- inkycal.custom.functions.auto_fontsize(font, max_height)
Scales a given font to 80% of max_height.
Gets the height of a font and scales it until 80% of the max_height is filled.
- Args:
font: A PIL Font object.
max_height: An integer representing the height to adjust the font to which the given font should be scaled to.
- Returns:
A PIL font object with modified height.
- inkycal.custom.functions.draw_border(image: <module 'PIL.Image' from '/home/runner/work/Inkycal/Inkycal/venv/lib/python3.11/site-packages/PIL/Image.py'>, xy: ~typing.Tuple[int, int], size: ~typing.Tuple[int, int], radius: int = 5, thickness: int = 1, shrinkage: ~typing.Tuple[int, int] = (0.1, 0.1)) None
Draws a border at given coordinates.
- Args:
image: The image on which the border should be drawn (usually im_black or im_colour).
xy: Tuple representing the top-left corner of the border e.g. (32, 100) where 32 is the x-coordinate and 100 is the y-coordinate.
size: Size of the border as a tuple -> (width, height).
radius: Radius of the corners, where 0 = plain rectangle, 5 = round corners.
thickness: Thickness of the border in pixels.
shrinkage: A tuple containing decimals presenting a percentage of shrinking -> (width_shrink_percentage, height_shrink_percentage). e.g. (0.1, 0.2) ~ shrinks the width of border by 10%, shrinks height of border by 20%
- inkycal.custom.functions.get_fonts()
Print all available fonts by name.
Searches the /font folder in Inkycal and displays all fonts found in there.
- Returns:
printed output of all available fonts. To access a fontfile, use the fonts dictionary to access it.
>>> fonts['fontname']
To use a font, use the following sytax, where fontname is one of the printed fonts of this function:
>>> ImageFont.truetype(fonts['fontname'], size = 10)
- inkycal.custom.functions.get_system_tz() str
Gets the system-timezone
Gets the timezone set by the system.
- Returns:
A timezone if a system timezone was found.
UTC if no timezone was found.
The extracted timezone can be used to show the local time instead of UTC. e.g.
>>> import arrow >>> print(arrow.now()) # returns non-timezone-aware time >>> print(arrow.now(tz=get_system_tz())) # prints timezone aware time.
- inkycal.custom.functions.internet_available() bool
checks if the internet is available.
Attempts to connect to google.com with a timeout of 5 seconds to check if the network can be reached.
- Returns:
True if connection could be established.
False if the internet could not be reached.
Returned output can be used to add a check for internet availability:
>>> if internet_available(): >>> #...do something that requires internet connectivity
- inkycal.custom.functions.text_wrap(text: str, font=None, max_width=None)
Splits a very long text into smaller parts
Splits a long text to smaller lines which can fit in a line with max_width. Uses a Font object for more accurate calculations.
- Args:
text -> Text as a string
font: A PIL font object which is used to calculate the size.
max_width: int-> a width in pixels defining the maximum width before splitting the text into the next chunk.
- Returns:
A list containing chunked strings of the full text.
- inkycal.custom.functions.write(image: <module 'PIL.Image' from '/home/runner/work/Inkycal/Inkycal/venv/lib/python3.11/site-packages/PIL/Image.py'>, xy: ~typing.Tuple[int, int], box_size: ~typing.Tuple[int, int], text: str, font=None, **kwargs)
Writes text on an image.
Writes given text at given position on the specified image.
- Args:
image: The image to draw this text on, usually im_black or im_colour.
xy: tuple-> (x,y) representing the x and y co-ordinate.
box_size: tuple -> (width, height) representing the size of the text box.
text: string, the actual text to add on the image.
font: A PIL Font object e.g. ImageFont.truetype(fonts[‘fontname’], size = 10).
- Args: (optional)
alignment: alignment of the text, use ‘center’, ‘left’, ‘right’.
autofit: bool (True/False). Automatically increases fontsize to fill in as much of the box-height as possible.
colour: black by default, do not change as it causes issues with rendering on e-Paper.
rotation: Rotate the text with the text-box by a given angle anti-clockwise.
fill_width: Decimal representing a percentage e.g. 0.9 # 90%. Fill maximum of 90% of the size of the full width of text-box.
fill_height: Decimal representing a percentage e.g. 0.9 # 90%. Fill maximum of 90% of the size of the full height of the text-box.
Helper classes
Inkycal iCalendar parsing module Copyright by aceinnolab
- class inkycal.modules.ical_parser.iCalendar
iCalendar parsing moudule for inkycal. Parses events from given iCalendar URLs / paths
- static all_day(event)
Check if an event is an all day event. Returns True if event is all day, else False
- clear_events()
clear previously parsed events
- get_events(timeline_start, timeline_end, timezone=None)
Input an arrow (time) object for: * the beginning of timeline (events have to end after this time) * the end of the timeline (events have to begin before this time) * timezone if events should be formatted to local time Returns a list of events sorted by date
- static get_system_tz()
Get the timezone set by the system
- load_from_file(filepath)
Input a string or list of strings containing valid iCalendar filepaths example: ‘path1’ (single file) OR [‘path1’, ‘path2’] (multiple files) returns a list of iCalendars as string (raw)
- load_url(url, username=None, password=None)
Input a string or list of strings containing valid iCalendar URLs example: ‘URL1’ (single url) OR [‘URL1’, ‘URL2’] (multiple URLs) add username and password to access protected files
- show_events(fmt='DD MMM YY HH:mm')
print all parsed events in a more readable way use the format (fmt) parameter to specify the date format see https://arrow.readthedocs.io/en/latest/#supported-tokens for more info tokens
- sort()
Sort all parsed events in order of beginning time
Custom image class for Inkycal Project Takes care of handling images. Made to be used by other modules to handle images.
Copyright by aceinnolab
- class inkycal.modules.inky_image.Inkyimage(image=None)
Custom Imgae class written for commonly used image operations.
- autoflip(layout: str) None
flips the image automatically to the given layout.
- Args:
layout:-> str. Choose horizontal or vertical.
Checks the image’s width and height.
In horizontal mode, the image is flipped if the image height is greater than the image width.
In vertical mode, the image is flipped if the image width is greater than the image height.
- clear()
Removes currently saved image if present.
- flip(angle)
Flips the image by the given angle.
- Args:
angle:->int. A multiple of 90, e.g. 90, 180, 270, 360.
- load(path: str) None
loads an image from a URL or filepath.
- Args:
path:The full path or url of the image file e.g. https://sample.com/logo.png or /home/pi/Downloads/nice_pic.png
- Raises:
FileNotFoundError: This Exception is raised when the file could not be found.
OSError: A OSError is raised when the URL doesn’t point to the correct file-format, i.e. is not an image
TypeError: if the URLS doesn’t start with htpp
- static merge(image1, image2)
Merges two images into one.
Replaces white pixels of the first image with transparent ones. Then pastes the first image on the second one.
- Args:
image1: A PIL Image object in ‘RGBA’ mode.
image2: A PIL Image object in ‘RGBA’ mode.
- Returns:
A single image.
- remove_alpha()
Removes transparency if image has transparency.
Checks if an image has an alpha band and replaces the transparency with white pixels.
- resize(width=None, height=None)
Resize an image to desired width or height
- inkycal.modules.inky_image.image_to_palette(image: <module 'PIL.Image' from '/home/runner/work/Inkycal/Inkycal/venv/lib/python3.11/site-packages/PIL/Image.py'>, palette: ~typing.Literal = ['bwr', 'bwy', 'bw', '16gray'], dither: bool = True) -> (<module 'PIL.Image' from '/home/runner/work/Inkycal/Inkycal/venv/lib/python3.11/site-packages/PIL/Image.py'>, <module 'PIL.Image' from '/home/runner/work/Inkycal/Inkycal/venv/lib/python3.11/site-packages/PIL/Image.py'>)
Maps an image to a given colour palette.
Maps each pixel from the image to a colour from the palette.
- Args:
palette: A supported token. (see below)
dither:->bool. Use dithering? Set to False for solid colour fills.
- Returns:
two images: one for the coloured band and one for the black band.
- Raises:
ValueError if palette token is not supported
Supported palette tokens:
>>> 'bwr' # black-white-red >>> 'bwy' # black-white-yellow >>> 'bw' # black-white >>> '16gray' # 16 shades of gray